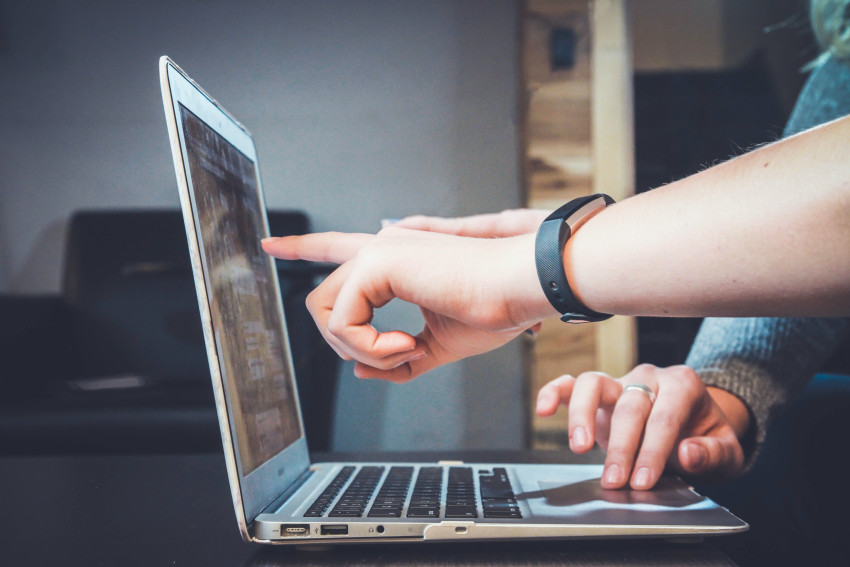
In today's world, developers are increasingly working on cross-platform applications that can run on various devices, operating systems, and cloud platforms. Writing portable .NET code that can run on different platforms can be a challenging task, but it's an essential skill for any .NET developer. Here are some tips for writing portable .NET code.
Use Platform Abstractions
One of the most important things to keep in mind when writing portable .NET code is to use platform abstractions. Instead of using platform-specific APIs directly, use the appropriate abstraction layer. For example, instead of using the System.IO.File class, use the IFile abstraction provided by the Microsoft.Extensions.FileProviders.Abstractions package. This will make your code more portable and easier to maintain.
Use Cross-Platform Libraries
Using cross-platform libraries can significantly simplify the task of writing portable .NET code. There are several popular cross-platform libraries available that can help you build applications that can run on multiple platforms. Some popular cross-platform libraries include Newtonsoft.Json for JSON serialization, RestSharp for REST API clients, and NUnit for unit testing.
Avoid Platform-Specific Code
Avoid using platform-specific code in your application logic as much as possible. For example, don't use Windows-specific APIs in your code if you want to run it on Linux or macOS. Instead, use cross-platform abstractions, as discussed earlier.
Use Dependency Injection
Dependency injection can be a powerful tool for writing portable .NET code. By using a dependency injection framework, you can abstract away platform-specific details and make your code more portable. Dependency injection frameworks such as Microsoft.Extensions.DependencyInjection can help you manage dependencies and reduce coupling between components.
Use .NET Standard
.NET Standard is a specification that defines a set of APIs that must be implemented by all .NET platforms that support the standard. By targeting .NET Standard instead of a specific platform, you can ensure that your code will run on any platform that supports .NET Standard. When writing portable .NET code, it's a good idea to target the highest version of .NET Standard that is compatible with all the platforms you're targeting.
Test Your Code on Multiple Platforms
To ensure that your code is truly portable, you should test it on multiple platforms. Use a continuous integration and delivery (CI/CD) system to automatically build and test your code on various platforms. This will help you catch platform-specific issues early in the development process and ensure that your code is truly portable.
Follow Best Practices
Finally, follow best practices when writing portable .NET code. Use meaningful variable names, write clear and concise code, and follow established design patterns and principles. By following best practices, you can ensure that your code is maintainable, easy to read, and of high quality.
In conclusion, writing portable .NET code is an essential skill for any hire dot NET developer. By using platform abstractions, cross-platform libraries, avoiding platform-specific code, using dependency injection, targeting .NET Standard, testing on multiple platforms, and following best practices, you can write portable .NET code that can run on multiple platforms.
Use Portable Libraries
Portable libraries are an excellent way to write code that can be shared across different .NET platforms. Portable Class Libraries (PCLs) were introduced in .NET Framework 4.0 and provide a way to write libraries that can be used in different .NET platforms such as Windows, Windows Phone, and Xbox.
When creating a portable library, it is essential to choose the correct set of platforms you want to target. Choosing too many platforms can make it difficult to write code that works well across all of them. It is also important to avoid using any platform-specific APIs or libraries.
Use Conditional Compilation
Conditional compilation is a powerful feature in .NET that allows you to include or exclude code based on specific conditions. This can be useful when you need to write code that works differently on different platforms.
For example, you might want to include specific code for Windows or exclude code that is not supported on a specific version of .NET. By using conditional compilation, you can write code that works on multiple platforms without having to create separate projects or libraries.
Use NuGet Packages
NuGet is a package manager for .NET that allows you to easily install and manage packages for your projects. NuGet packages can contain code, binaries, libraries, and other resources that you can use in your projects.
Using NuGet packages can save you a lot of time and effort, as you can easily add functionality to your projects without having to write everything from scratch. It also helps to ensure that your code is portable, as NuGet packages are designed to work across different .NET platforms.
Avoid Platform-Specific APIs
It is essential to avoid using platform-specific APIs when writing portable .NET code. These APIs are designed to work on a specific platform and may not be available on other platforms.
For example, if you are writing code that needs to access the file system, you should use the System.IO namespace instead of platform-specific APIs. This will ensure that your code can be used on any platform that supports .NET.
Test Your Code on Multiple Platforms
Testing your code on multiple platforms is essential to ensure that it is truly portable. It is important to test your code on all the platforms that you are targeting, as well as any additional platforms that you may want to support in the future.
This can be a time-consuming process, but it is essential to ensure that your code works correctly on all platforms. It is also important to test your code on different versions of .NET, as some features may be available in one version but not in another.
Conclusion
Writing portable .NET code is essential for creating robust and flexible applications. By following these tips, you can write code that can be easily shared across different platforms, making it easier to maintain and update your projects.
By using design patterns, conditional compilation, and portable libraries, you can create code that is efficient, scalable, and easy to maintain. It is also important to test your code on multiple platforms to ensure that it works correctly on all the platforms that you are targeting. By doing so, you can ensure that your code is truly portable and can be used by a wide range of users.